DOM Manipulation
DOM stands for Document Object Model. We can change and modify the content of our web document (which is simply the website displayed in the browser) through JavaScript code, without making any changes to our .html file.
Here are some of the ways that we can do it.
JavaScript Event Attributes
JavaScript Events are actions that you can assign to certain events that occur in HTML pages.
Here's how it works.
Let's select an element that we want to react on. We can give the element an event attribute inserting it directly inside the tag.
.html
<button id="button">Click Me!</button>
OR
<button id="button" onclick="functionName()">Click Me!</button>
.js
const button = document.getElementById('button');
function functionName(){
alert('Hello')
}
We can allso assign a function to our button in the .js file. In this case we only use the function name - we don't need to call the function with the parentheses.
button.onclick = functionName;
The full list of HTML Events can be found on w3schools.
Some of the most frequently used events include onclick, onmouseover, onmouseout, onmousedown, onmouseup, onload and onchange. Let's see them in action.
First, I will declare some functions.
function redFont() {
button.style.color = "red"
}
function greenFont() {
button.style.color = "green"
}
function yellowFont(){
button.style.color = "yellow"
}
function blackFont(){
button.style.color = "black"
}
Now let's use these functions as events on our button. When you hover over it the colour of the
font should change to red.
Note that the font will stay red even when the mouse pointer leaves the button.
button.onmouseover = redFont;
Let's tell our button what to do when the mouse stops hovering over it - we will change the color of the font to green.
button.onmouseout = greenFont;
Let's change the colour of the font to yellow when we click the button.
Note that the font stays
yellow even after the button has been clicked.
button.onmousedown = yellowFont;
Now let's change the color of the font back to black, when the click is finished.
button.onmouseup = blackFont;
Let's trigger an alert when you make a change to an input.
Note that you need to click outside
of the input to trigger the event. The alert should pop up after every change.
const input = document.getElementById('input')
function onChangeEvent() {
alert('You made a change!')
}
input.onchange = onChangeEvent;
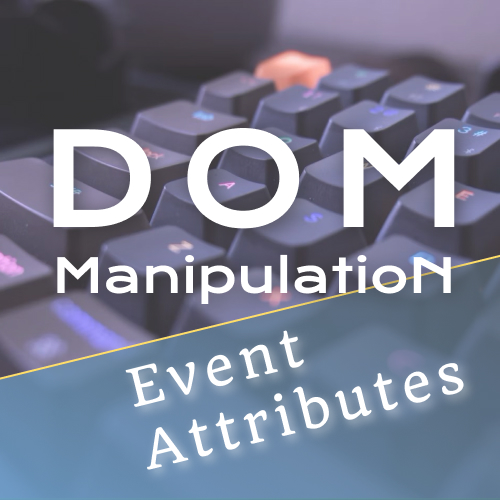
Comments (0)
Be the first to leave a comment